How to Connect an ORP Sensor to a Raspberry Pi
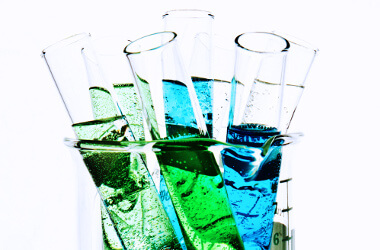
Knowing the chlorine level of your pool is very important in keeping your pool safe and sanitary. When it comes to pool chemistry though it is the free chlorine level that really matters. Your free chlorine level is what is actually killing the bacteria in your pool and should be maintained at between 1-3 ppm. The free chlorine level can be affected by many factors such as how much the pool is being used, the amount of stabilizer (cyanuric acid) and the pH of the water.
ORP is the acronym for Oxidation Reduction (REDOX) Potential, ORP sensors give the most accurate picture of the effect of all oxidizing and reducing chemicals in your pool. No in-depth knowledge or training is required to obtain accurate repeatable results. User error is virtually eliminated because ORP readings require no subjective, visual interpretation. The World Health Organization (WHO) suggests an ORP value of between 650-720 mV for safe swimming pool water.
The Atlas Scientific ORP sensor is an industrial grade sensor that works well with the Raspberry Pi, it is fully submersible up to the BNC connector in both fresh and saltwater. The sensor can work in serial or via the I2C protocol, for this project you will be configuring the sensor to use the I2C interface on the Pi.
To configure the Pi I am assuming that you are running the latest version of Raspbian and have the ability to connect to your Pi either through SSH with putty and FTP with Filezilla, or directly with a keyboard and monitor if you haven’t set-up your RPi yet then check out my getting started section.
Materials
In this tutorial I will be using the following materials:
- Raspberry Pi (2, 3 or 4)
- Micro SD Card
- Power Supply
- Atlas Scientific ORP Sensor Kit
- Breadboard
- Jumper Wires
- Adafruit T-Cobbler Plus (Optional)
- Raspberry Pi Case (Optional)
The first thing we need to do is enable the I2C modules on the Pi. This is done by entering the following at the command prompt to start the configuration tool
sudo raspi-config
select option 9 – Advanced Options
select option A7 – I2C
select “Yes” for all the questions and reboot the Pi
sudo reboot
After the reboot ensure that all of the Raspbian packages are up to date by running the following commands.
sudo apt update
sudo apt dist-upgrade
sudo apt autoremove
sudo apt clean
Next, check/add the i2c tools package.
sudo apt install i2c-tools
i2cdetect -y 1
This should produce the following without the sensor attached.
Now that we have our I2C module working correctly we can go ahead and connect our ORP sensor.
When describing the physical pin connections I will be following the GPIO pin numbering convention shown below.
Firstly we need to get the ORP circuit into the correct mode, when delivered the ORP circuit will be in UART (serial) mode, the ORP circuit has to be manually switched from UART mode to I2C mode. When this is done the ORP circuit will have its I2C address set to 98 (0x62 Hexadecimal).
Using your breadboard perform the following actions
- Cut the power to the device
- Disconnect any jumper wires going from TX and RX to the Pi
- Short the PGND pin to the TX pin
- Power the device
- Wait for LED to change from Green to Blue
- Remove the short from the probe pin to the TX pin
- Power cycle the device
The device is now I2C mode.
The Pi and ORP circuits are now configured so we can go ahead and connect it all together.
Assuming that all of the parts are now mounted on your breadboard
- Connect the GND pin of the ORP circuit to the ground pin of your Pi.
- Connect the TX(SDA) pin to GPIO pin 2.
- Connect the RX(SCL) pin to GPIO pin 3.
Note: Do Not Use jumper wires for these connections or your readings will not be accurate.
- The PRB and PGND pins should be connected via your breadboard to the center and shield pins of your BNC connector.
- Finally, power your ORP circuit by connecting the Vcc pin to the +3.3V pin.
You can now run a quick test to prove that we are set up correctly, from the command prompt enter the following:
i2cdetect -y 1
you should see the following response, if not then check your connections, ensure the light on the ORP circuit is blue and reboot your Pi.
In the image above I have 3 sensors connected to my Pi, the ORP sensor connection is indicated by Hex value 62. The factory pre-set for the ORP sensor is 98 or 62 in hexadecimal as mentioned above, if you have more than 1 ORP circuit connected then you will need to specify a different value. To do this we need to add some python code to our Pi.
Atlas Scientific provides the python code that I will be using here for interfacing with the ORP Circuit.
We start by importing the required python modules
#!/usr/bin/env python
import io # used to create file streams
import fcntl # used to access I2C parameters like addresses
import time # used for sleep delay and timestamps
import string # helps parse
Next, we add the class code to interface with the ORP circuit (or any other Atlas Scientific circuit for that matter)
class atlas_i2c:
long_timeout = 1.5 # the timeout needed to query readings and
#calibrations
short_timeout = .5 # timeout for regular commands
default_bus = 1 # the default bus for I2C on the newer Raspberry Pis,
# certain older boards use bus 0
default_address = 98 # the default address for the ORP sensor
def __init__(self, address=default_address, bus=default_bus):
# open two file streams, one for reading and one for writing
# the specific I2C channel is selected with bus
# it is usually 1, except for older revisions where its 0
# wb and rb indicate binary read and write
self.file_read = io.open("/dev/i2c-" + str(bus), "rb", buffering=0)
self.file_write = io.open("/dev/i2c-" + str(bus), "wb", buffering=0)
# initializes I2C to either a user specified or default address
self.set_i2c_address(address)
def set_i2c_address(self, addr):
# set the I2C communications to the slave specified by the address
# The commands for I2C dev using the ioctl functions are specified in
# the i2c-dev.h file from i2c-tools
I2C_SLAVE = 0x703
fcntl.ioctl(self.file_read, I2C_SLAVE, addr)
fcntl.ioctl(self.file_write, I2C_SLAVE, addr)
def write(self, string):
# appends the null character and sends the string over I2C
string += "